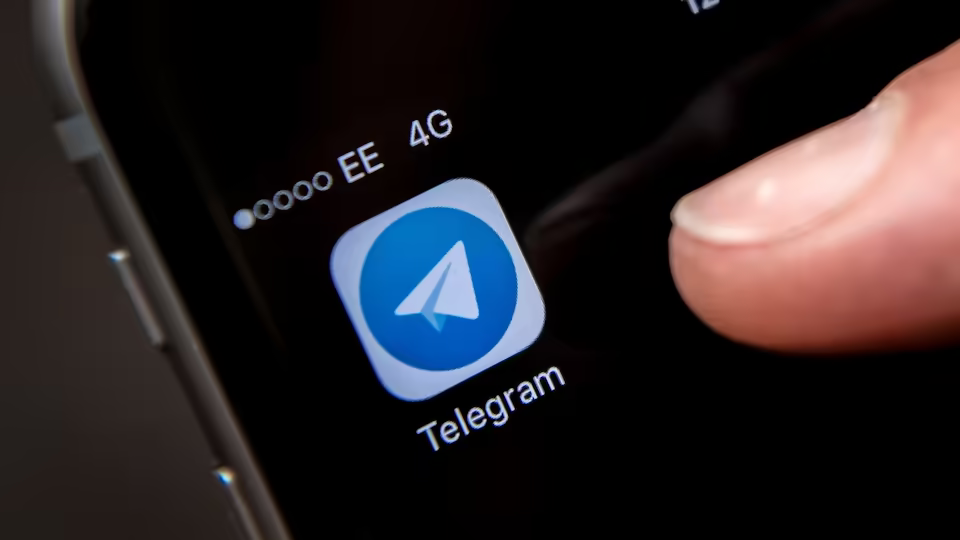
Table of contents
The problem
I was looking for a way to embed Telegram posts in my Svelte web application but couldn’t find any package in npm that does this, so I created my own.
Telegram posts
Telegram has channels and groups that can be private or public.
For the public, each post can be shared publicly using a unique URL in this format: https://t.me/{channel_name}/{post_id}
.
To be able to get an HTML embedded post preview in your website, you need to copy the URL by clicking/tapping Copy post link
in the context menu of that post.
After that you can you open this widget creator made by Telegram, paste your post URL and you’ll see a preview of the post.
There are other configurations for that preview explained here:
- Width:
This is the width of the embedded post, measured by percentage. The default value is
100%
. - Author photo:
Whether to show the author’s photo. The default value is
auto
, which means that the author’s photo will be shown if there’s enough width. - Accent color:
The color of the post’s accent. The default value is
#2481cc
. - Accent color in dark theme:
The color of the post’s accent in dark theme. The default value is
#2481cc
. - Dark theme:
Whether to use dark theme. The default value is
false
.
The way it works is to add a script to your website that loads the post preview from telegram server in the form of an iframe.
Discussions widget
Telegram also provides another widget to display comments on a post, while allowing your website users to engage with your posts after logging in to Telegram.
If you have a public channel and enabled discussions, you can get the post link and paste it in the widget creator.
Like post widget, the discussions widget also has some configurations.
- Comments limit:
The initial number of comments to display. The default value is
5
. - Height: This is the height of the embedded discussions widget, measured by pixels. The default value is set automatically by the telegram script depending on how many comments are displayed.
- Accent color:
The color of the widgets’ accent. The default value is
#2481cc
. - Accent color in dark theme:
The color of the widgets’ accent in dark theme. The default value is
#2481cc
. - Colorful names:
Whether to use different colors for usernames. The default value is
false
. - Dark theme:
Whether to use dark theme. The default value is
false
.
To add it to your website, you’ll do the same steps as post widget.
Adding those widgets in Svelte
In svelte, you can use only one <script>
tag in each component, that script tag contains all logic for that component.
So for adding an embedded Telegram post, you need to create a new DOM script element and add it to one of your elements that exists in your component, and set its source and other attributes according to the script tag you copied from Telegram widget creator.
This is a boring task, that’s why I made Sveltegram.
Sveltegram
I made this component to easily add embedded posts previews in your website.
This component is reactive as all svelte components should be, which means it will update the preview whenever the data changes, like if your website has a theme switch, you can bind that to the darkMode
property of this component and it will update the preview accordingly.
To view the demo, you can check out the webpage.
Installing
Using npm
, you can install it with this command:
npm install sveltegram
If you are using yarn or pnpm, you can install it with this command:
yarn add sveltegram
## Or
pnpm add sveltegram
Usage
Post widget
The very basic usage only requires the post link that you can copy from Telegram’s context menu.
<script>
import { Post } from 'sveltegram';
</script>
<Post link="https://t.me/computly/190" />
And the preview would be like this:
Testing dark mode:
<script>
import { Post } from 'sveltegram';
</script>
<Post link="https://t.me/computly/190" darkMode={true} />
Of course all props including dark mode are reactive so you can bind them to other variables.
There are other props like accent color and accent color in dark mode, you can try them in this demo.
Discussions widget
The basic usage is like this:
<script>
import { Discussions } from 'sveltegram';
</script>
<Discussions
link="https://t.me/contest/198"
color="#2f81f6"
colorDark="#89baff"
commentsLimit={5}
/>
Just like post widget, all props are reactive so you can bind them to other variables.
You can test dark mode and other props in this demo.